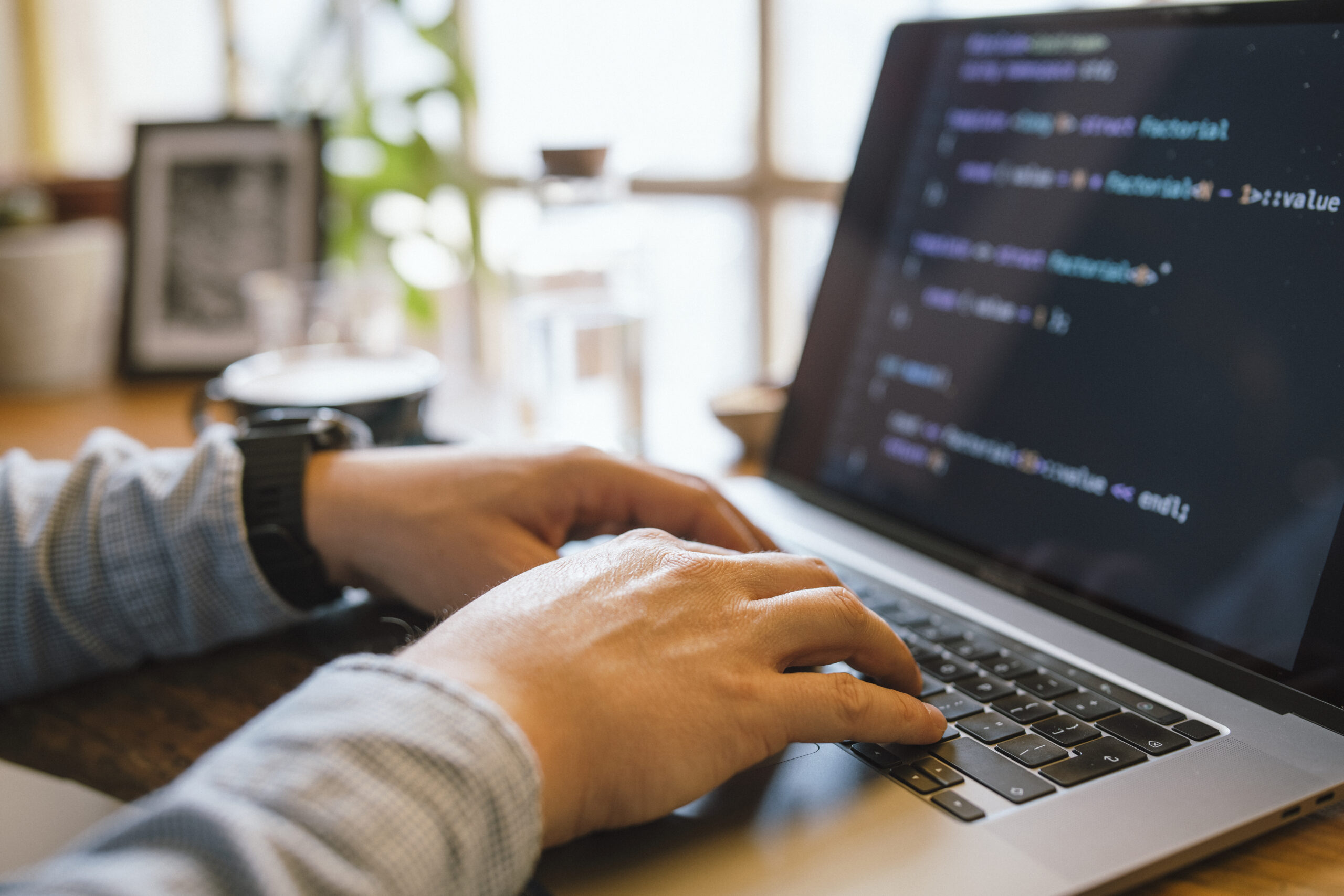
Debugging is One of the more vital — nonetheless often disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about knowing how and why factors go Mistaken, and Mastering to Imagine methodically to unravel complications efficiently. Whether or not you're a beginner or a seasoned developer, sharpening your debugging abilities can save hours of aggravation and drastically boost your productivity. Listed below are numerous methods to assist developers amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of several quickest methods builders can elevate their debugging techniques is by mastering the instruments they use every single day. Although creating code is 1 part of enhancement, recognizing tips on how to communicate with it successfully throughout execution is Similarly vital. Modern-day progress environments appear Outfitted with strong debugging capabilities — but many builders only scratch the floor of what these instruments can do.
Consider, for instance, an Built-in Progress Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications help you set breakpoints, inspect the worth of variables at runtime, phase via code line by line, as well as modify code over the fly. When utilised appropriately, they Permit you to notice particularly how your code behaves in the course of execution, which is a must have for tracking down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclusion developers. They allow you to inspect the DOM, watch network requests, perspective actual-time performance metrics, and debug JavaScript while in the browser. Mastering the console, sources, and community tabs can change disheartening UI troubles into manageable jobs.
For backend or program-stage developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management more than jogging processes and memory administration. Understanding these applications may have a steeper Mastering curve but pays off when debugging overall performance challenges, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into comfortable with Edition Regulate systems like Git to grasp code record, discover the exact instant bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your instruments suggests likely further than default settings and shortcuts — it’s about developing an intimate understanding of your progress environment in order that when troubles occur, you’re not shed at midnight. The better you understand your equipment, the more time you'll be able to commit resolving the particular challenge rather then fumbling as a result of the procedure.
Reproduce the condition
One of the most critical — and infrequently overlooked — actions in efficient debugging is reproducing the issue. In advance of leaping in the code or building guesses, builders will need to produce a regular surroundings or situation exactly where the bug reliably appears. Without reproducibility, fixing a bug will become a match of likelihood, frequently bringing about squandered time and fragile code adjustments.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire thoughts like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it turns into to isolate the precise problems under which the bug takes place.
When you’ve gathered adequate information and facts, try and recreate the problem in your neighborhood environment. This might suggest inputting a similar info, simulating similar consumer interactions, or mimicking system states. If The problem seems intermittently, contemplate crafting automated assessments that replicate the edge situations or point out transitions concerned. These assessments not only aid expose the condition but additionally reduce regressions in the future.
Often, The difficulty might be setting-unique — it might come about only on sure operating programs, browsers, or underneath particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But as soon as you can consistently recreate the bug, you're presently halfway to repairing it. By using a reproducible circumstance, You may use your debugging applications more successfully, check prospective fixes securely, and talk far more Plainly using your staff or people. It turns an summary grievance into a concrete challenge — and that’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Improper. As opposed to seeing them as irritating interruptions, builders really should study to deal with error messages as immediate communications within the process. They typically let you know just what happened, where it transpired, and from time to time even why it took place — if you know how to interpret them.
Begin by reading the information meticulously and in full. Lots of builders, especially when less than time force, glance at the first line and promptly start off creating assumptions. But further while in the error stack or logs may well lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google — browse and realize them initial.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or simply a logic error? Will it stage to a selected file and line amount? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can substantially increase your debugging procedure.
Some glitches are imprecise or generic, and in People conditions, it’s essential to look at the context wherein the error transpired. Test associated log entries, input values, and up to date changes within the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about possible bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint challenges faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When employed efficiently, it provides actual-time insights into how an application behaves, aiding you recognize what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging ranges contain DEBUG, Information, WARN, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic info throughout improvement, Details for normal gatherings (like thriving start out-ups), WARN for possible issues that don’t crack the applying, ERROR for actual problems, and Lethal once the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your technique. Concentrate on key situations, condition modifications, enter/output values, and demanding choice details within your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance click here names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting This system. They’re especially worthwhile in production environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-assumed-out logging method, you may reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and trustworthiness within your code.
Believe Just like a Detective
Debugging is not merely a technical activity—it's a sort of investigation. To effectively determine and correct bugs, builders will have to method the process just like a detective resolving a mystery. This frame of mind can help stop working complicated issues into manageable elements and observe clues logically to uncover the foundation result in.
Start off by accumulating proof. Look at the signs and symptoms of the challenge: mistake messages, incorrect output, or general performance problems. Similar to a detective surveys a criminal offense scene, obtain just as much relevant details as it is possible to without the need of jumping to conclusions. Use logs, test circumstances, and consumer stories to piece alongside one another a transparent photograph of what’s going on.
Next, form hypotheses. Ask your self: What could be creating this behavior? Have any alterations not long ago been manufactured on the codebase? Has this situation occurred prior to under similar instances? The objective would be to narrow down alternatives and detect probable culprits.
Then, examination your theories systematically. Make an effort to recreate the issue in a managed surroundings. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Similar to a detective conducting interviews, check with your code queries and Enable the final results lead you nearer to the truth.
Pay near interest to compact facts. Bugs usually disguise while in the least predicted areas—similar to a missing semicolon, an off-by-a person error, or simply a race issue. Be thorough and individual, resisting the urge to patch the issue with no totally knowledge it. Short-term fixes may well hide the true problem, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging process can preserve time for long term problems and enable Other people fully grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical abilities, strategy complications methodically, and grow to be simpler at uncovering concealed issues in sophisticated devices.
Write Exams
Composing checks is among the most effective approaches to transform your debugging skills and General advancement effectiveness. Checks don't just assist catch bugs early but in addition function a security Internet that provides you self esteem when making modifications for your codebase. A properly-analyzed software is much easier to debug because it permits you to pinpoint just wherever and when a dilemma takes place.
Get started with device checks, which deal with individual capabilities or modules. These small, isolated checks can quickly reveal regardless of whether a particular piece of logic is Operating as expected. When a test fails, you immediately know where to look, significantly lessening some time expended debugging. Unit tests are Particularly useful for catching regression bugs—issues that reappear just after Earlier currently being set.
Subsequent, combine integration assessments and stop-to-stop tests into your workflow. These assistance be sure that several areas of your application do the job jointly easily. They’re especially useful for catching bugs that come about in intricate methods with multiple parts or solutions interacting. If a little something breaks, your exams can tell you which Component of the pipeline failed and less than what situations.
Writing assessments also forces you to Consider critically about your code. To check a function adequately, you will need to be familiar with its inputs, anticipated outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging an issue, composing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you may concentrate on repairing the bug and view your examination go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—encouraging you catch a lot more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after Alternative. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind results in being fewer economical at trouble-resolving. A brief stroll, a coffee crack, or even switching to another endeavor for ten–quarter-hour can refresh your emphasis. A lot of developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, Specially in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You would possibly out of the blue notice a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you right before.
In case you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all-around, stretch, or do a thing unrelated to code. It might sense counterintuitive, Specifically less than tight deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise system. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of resolving it.
Learn From Just about every Bug
Every bug you come across is a lot more than just A brief setback—It is really an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural issue, each one can train you a little something valuable should you make the effort to replicate and analyze what went Incorrect.
Commence by inquiring on your own a handful of key concerns once the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with improved tactics like unit testing, code reviews, or logging? The answers often reveal blind places in your workflow or understanding and assist you to Develop stronger coding habits shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring difficulties or prevalent problems—which you can proactively stay away from.
In team environments, sharing what you've acquired from the bug using your peers may be Particularly powerful. Irrespective of whether it’s via a Slack concept, a short produce-up, or a quick understanding-sharing session, encouraging Some others stay away from the same difficulty boosts staff efficiency and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the best builders aren't those who create great code, but people who consistently understand from their errors.
Eventually, Each and every bug you repair provides a fresh layer to your ability established. So up coming time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging skills will take time, exercise, and tolerance — nevertheless the payoff is big. It will make you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at That which you do.